Laravel : Images compress with intervention v3.0
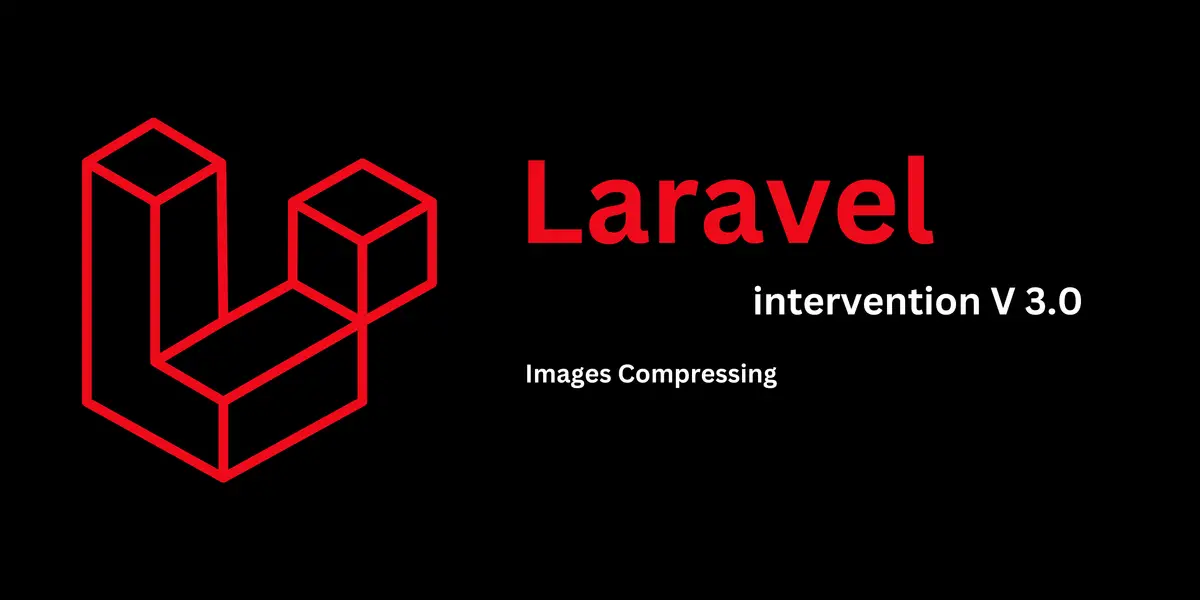
Every framework and programming languages have image manipulation libraries or tools. In Laravel we can introduce the Intervention library. It was built on top of the GD library or ImageMagick library. When we install PHP GD library is automatically implemented into the system. Therefore we don't need to worry about that.
Intervention is an open source and developers can compress images, crop, change height and width, etc. Intervention Image released two versions v2 and v3. In this blog, we are planning to explain about the V3. Let's discuss that,
Why should images compress
- Large-size images are not rendering fast on the server.
- A compressed image takes less time for uploading images to form submission.
- Another main reason is we can save more storage space in the web hosting or HDD drivers.
- Large-size images can interrupt to system and significantly aid in system failures.
- Compressed images always provide better UI/UX to the user.
Features
- Easy manipulation process of images
- Well-improved code architecture
- Suport for GD & Imagick libaries
- Open-Source
Server Requirements
- You have already installed an image processing library like GD Image or ImageMagick -- Hint: you can find it from Package.lock file
- Mbstring PHP extension
- PHP version >=8.1
Installation
For installing the Intervention library with composer try the scenario below command.
If it's successfully installation, composer.json will be updated automatically. In this scenario, we are planning to reduce the image size before storing it storage/app/public. When images are saved on the mentioned path. First, we have to install the below symlink.
We need to create a route file on web.php.
routes/web.php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\UserController;
Route::post('userStore', [UserController::class, 'userStore'])->name('user_store');
Then we have to create a controller to manipulate the image process. above web.php we have imported the userController.php. According to that, we create a public function for image-storing purposes.
Controllers/UserController.php
use App\Http\Controllers\Controller;
use Illuminate\Support\Facades\Storage;
use Intervention\Image\ImageManager;
use Intervention\Image\Drivers\Gd\Driver;
class UserController extends Controller {
public function userStore(Request $request){
try{
if ($request->file('file')) {
$image = $request->file('file');
$storePath = 'public/images';
$imageName = uniqid().'.'.$image->extension();
$image->storeAs($storePath,$imageName); //stored image
$storedPath = $manager->read("app/public/image/{$imageName}");
$manager = new ImageManager(new Driver());
//read Image
$image = $manager->read($storedPath);
$image = $image->scale(width:1024,height:780);
$image->toPng()->save($storedPath);
return response()->json(true);
}
} catch(\Throwable $th){
return response()->json(false);
}
}
}
In the above code snippet first, we have to store images in a given location in a normal way. Then we need to add the ImageManager method. Go through that method can be initialized to read images which previously stored. Collecting that image as the next step we have to set the scale. That can be initialized as we preferred scale. That should be the pixel (px) length unit. As the final step compressed image will be replaced with on previous existing image. that contains the method as toPng(). Don't worry to use that, it will never transform an image to PNG format. That is just a method and our original image extension will be kept.
Here, we can see the final output of the compression process. The left-hand side image is the original image. The right-hand side image is compressed. This example provides a clear idea about the compression process. How percentage image size was reduced. It may be 15x.
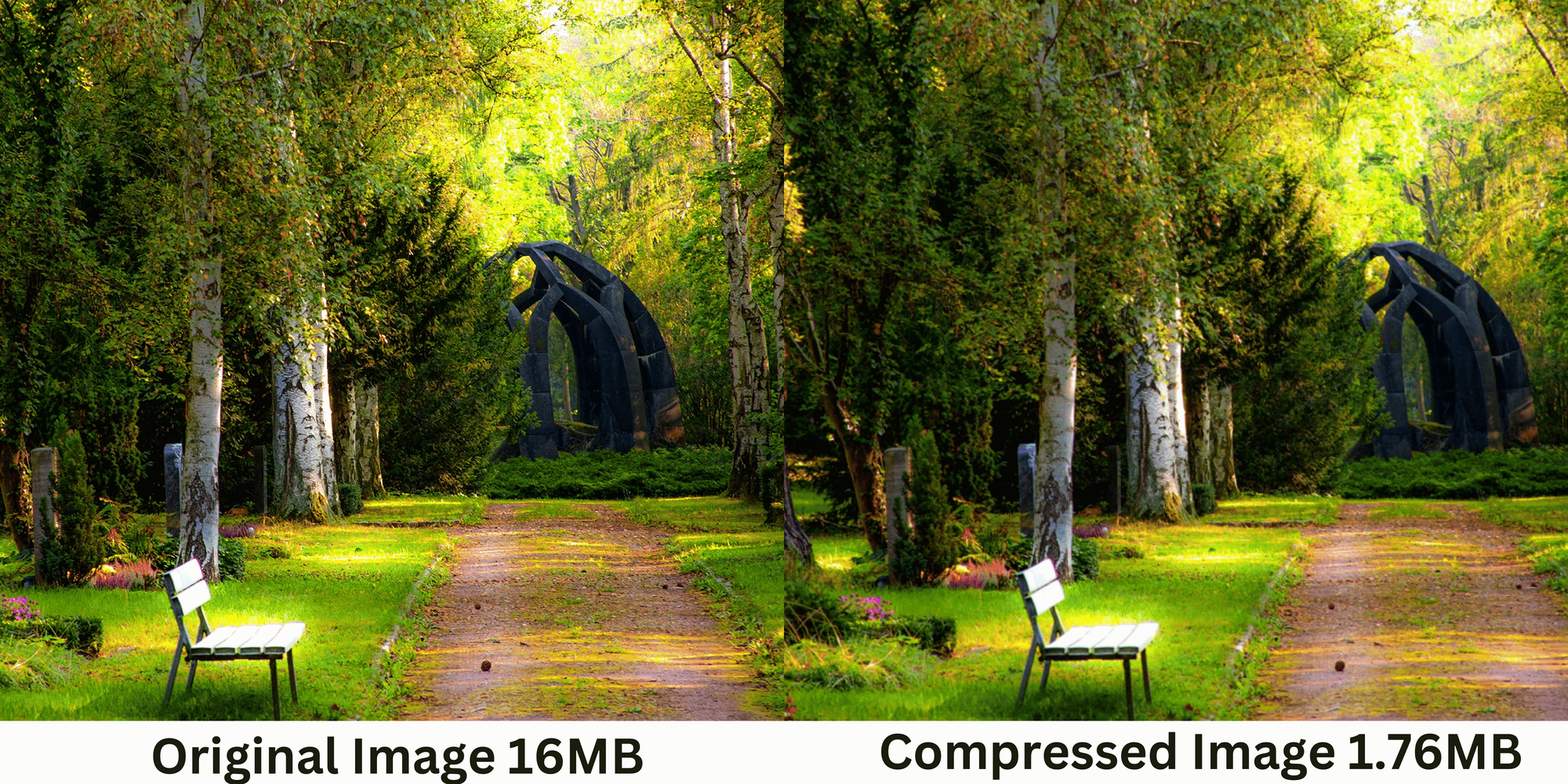